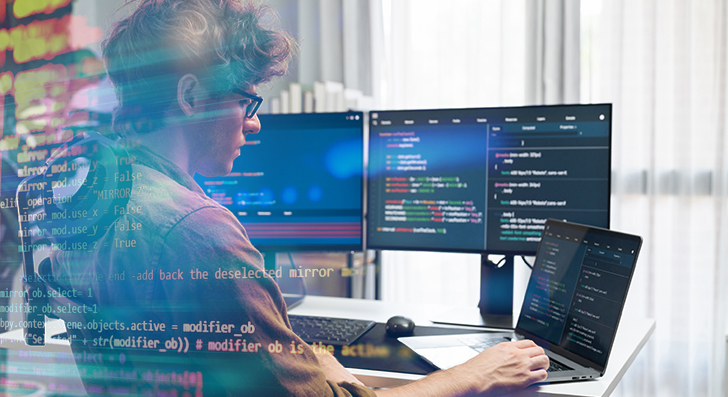
Scalability implies your application can manage development—more end users, a lot more information, and more targeted visitors—devoid of breaking. Like a developer, developing with scalability in your mind saves time and anxiety afterwards. Below’s a clear and realistic guidebook to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability is just not anything you bolt on afterwards—it should be part of the plan from the start. Many apps are unsuccessful every time they expand speedy due to the fact the first design and style can’t tackle the extra load. Being a developer, you need to think early about how your process will behave under pressure.
Start off by building your architecture for being versatile. Avoid monolithic codebases the place all the things is tightly linked. Instead, use modular design and style or microservices. These designs crack your app into more compact, impartial pieces. Every module or company can scale on its own with no influencing The complete method.
Also, take into consideration your databases from working day one. Will it require to take care of one million users or perhaps a hundred? Select the suitable style—relational or NoSQL—based on how your info will expand. Approach for sharding, indexing, and backups early, even if you don’t require them but.
One more significant place is to stay away from hardcoding assumptions. Don’t generate code that only works under recent problems. Contemplate what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use design and style patterns that aid scaling, like concept queues or function-driven systems. These support your app deal with much more requests without having receiving overloaded.
Any time you Establish with scalability in your mind, you are not just planning for achievement—you are lowering future headaches. A well-prepared procedure is less complicated to take care of, adapt, and grow. It’s much better to prepare early than to rebuild later.
Use the ideal Databases
Picking out the suitable databases can be a essential Section of constructing scalable programs. Not all databases are constructed a similar, and utilizing the Mistaken one can slow you down or even cause failures as your application grows.
Start off by knowledge your knowledge. Could it be very structured, like rows within a table? If Of course, a relational database like PostgreSQL or MySQL is a good fit. These are strong with interactions, transactions, and regularity. Additionally they assistance scaling procedures like examine replicas, indexing, and partitioning to deal with more targeted visitors and knowledge.
In case your details is much more flexible—like person action logs, item catalogs, or files—take into account a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling substantial volumes of unstructured or semi-structured information and will scale horizontally additional very easily.
Also, take into consideration your go through and generate designs. Are you presently undertaking many reads with fewer writes? Use caching and browse replicas. Are you currently dealing with a large write load? Investigate databases that can take care of superior create throughput, as well as celebration-based info storage programs like Apache Kafka (for short-term knowledge streams).
It’s also clever to Believe forward. You might not will need advanced scaling attributes now, but picking a databases that supports them suggests you won’t want to change later on.
Use indexing to hurry up queries. Prevent unnecessary joins. Normalize or denormalize your data according to your accessibility designs. And constantly watch database overall performance as you develop.
In brief, the proper database depends upon your app’s structure, velocity requires, And exactly how you anticipate it to develop. Take time to pick sensibly—it’ll help save many issues later on.
Optimize Code and Queries
Quick code is key to scalability. As your application grows, just about every compact hold off adds up. Badly created code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s crucial that you Construct effective logic from the beginning.
Start out by crafting cleanse, basic code. Stay away from repeating logic and remove nearly anything unneeded. Don’t choose the most complex Option if an easy 1 is effective. Maintain your features brief, concentrated, and simple to check. Use profiling equipment to locate bottlenecks—sites the place your code requires much too prolonged to run or works by using an excessive amount memory.
Up coming, evaluate your database queries. These normally sluggish things down a lot more than the code itself. Be sure Every question only asks for the data you really want. Stay clear of Pick *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specifically throughout big tables.
For those who recognize the exact same information currently being asked for again and again, use caching. Keep the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat high priced operations.
Also, batch your database functions after you can. Rather than updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with substantial datasets. Code and queries that work wonderful with a hundred documents could possibly crash once they have to deal with 1 million.
In a nutshell, scalable apps are rapidly applications. Keep the code limited, your queries lean, and use caching when desired. These ways aid your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more end users and a lot more website traffic. If all the things goes as a result of a person server, it'll rapidly become a bottleneck. That’s where load balancing and caching come in. Both of these instruments enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of a person server accomplishing the many operate, the load balancer routes consumers to distinct servers according to availability. This suggests no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other individuals. Resources like Nginx, HAProxy, or cloud-based options from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing info temporarily so it could be reused swiftly. When users ask for the identical info all over again—like a product page or simply a profile—you don’t ought to fetch it in the database anytime. You'll be able to provide it through the cache.
There are two prevalent kinds of caching:
one. Server-side caching (like Redis or Memcached) suppliers knowledge in memory for fast entry.
2. Customer-facet caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances velocity, and helps make your application a lot more economical.
Use caching for things that don’t transform frequently. And constantly make sure your cache is up-to-date when information does improve.
In brief, load balancing and caching are uncomplicated but potent equipment. Alongside one another, they help your app tackle much more end users, continue to be quick, and Get well from complications. If you plan to expand, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you may need applications that let your app expand quickly. That’s where by cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you'll need them. You don’t must acquire hardware or guess foreseeable future ability. When website traffic improves, you could increase more resources with just a few clicks or automatically making use of automobile-scaling. When site visitors drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You'll be able to give attention to creating your app instead of running infrastructure.
Containers are A further critical Resource. A container deals your app and every little thing it must run—code, libraries, configurations—into one particular unit. This makes it quick to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your app works by using various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single component within your app crashes, it restarts it automatically.
Containers also enable it to be simple to separate portions of your app into expert services. It is possible to update or scale parts independently, and that is great for general performance and dependability.
In brief, applying cloud and container equipment means you may scale quick, deploy quickly, and recover promptly when issues transpire. If you'd like your application to develop devoid of limits, start off applying these equipment early. They help you save time, decrease chance, and enable you to continue to be focused on creating, not correcting.
Monitor Almost everything
For those who don’t keep track of your software, you received’t know when things go Improper. Checking allows you see how your app is executing, location challenges early, and make much better choices as your application grows. It’s a vital Portion of making scalable units.
Begin by tracking standard metrics like CPU utilization, memory, disk House, and reaction time. These tell you how your servers and solutions are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this data.
Don’t just keep track of your servers—check your app also. Control just how long it will require for people to load web pages, how often errors happen, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly Gustavo Woltmann blog will help you see what’s happening inside your code.
Set up alerts for important issues. For instance, In case your response time goes above a Restrict or maybe a assistance goes down, it is best to get notified promptly. This will help you correct concerns quick, frequently before customers even discover.
Checking is likewise valuable once you make adjustments. In the event you deploy a new element and see a spike in mistakes or slowdowns, you can roll it again just before it leads to serious problems.
As your app grows, traffic and facts enhance. With out checking, you’ll overlook signs of issues until finally it’s also late. But with the right instruments in place, you continue to be in control.
To put it briefly, monitoring allows you maintain your application reputable and scalable. It’s not just about recognizing failures—it’s about comprehending your process and ensuring it really works nicely, even stressed.
Last Feelings
Scalability isn’t just for massive companies. Even modest apps want a solid foundation. By coming up with cautiously, optimizing sensibly, and using the suitable tools, it is possible to build apps that improve smoothly with no breaking stressed. Begin smaller, think huge, and Make intelligent.